Docker provides framework to isolate an application and its dependencies into self-contained unit called containers. These containers can be run anywhere. Containers can be considered as “light” version of virtual machines.
https://docs.docker.com/engine/installation
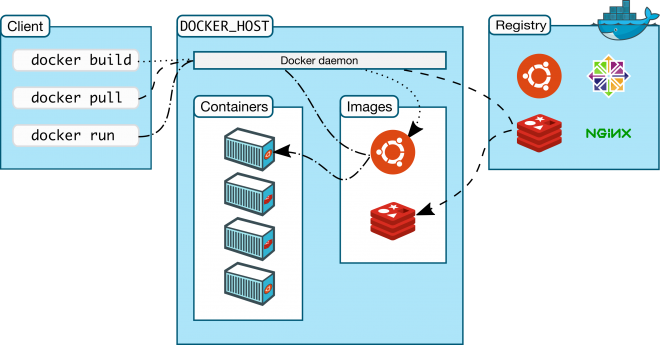
1
2
3
|
docker pull centos:6.8
docker pull ubuntu:14.04
docker pull centos # downloads latest image
|
1
2
3
4
5
|
# show running containers
docker ps
# show all containers (running and stopped)
docker ps -a
|
In detached mode, container runs in background as a daemon.
1
2
3
4
5
6
7
8
9
10
|
# detached mode, with pseudo-tty. if pseudo-tty is not used, the container
# will exit immediately after running as daemon
# with -d container keeps running in background as daemon
# -d Detached mode: Run container in the background
# -t Allocate a pseudo-tty
docker run -t -d centos:6.8
# specifying container memory
docker run -t -d --memory="1g" centos:6.8
|
In interactive mode, containers run in foreground attached to local command line session.
1
2
3
4
5
|
# interactive mode
# run container, attached interactively to local command line session and run /bin/bash
# in this case, the container exits when we exit from /bin/bash
docker run -i -t centos:6.8 /bin/bash
|
1
|
docker exec -it <container id or name> bash
|
1
|
docker stop <container id or name>
|
1
|
docker start <container id or name>
|
1
|
docker rm <container name or id>
|
1
|
docker run -t -d --name centos_container -p 80:80 centos:6.8
|
1
2
|
# -v /host/directory:/container/directory
docker run -i -t -v $(pwd):/opt centos:6.8 /bin/bash
|
1
|
docker inspect <container_id> | grep IPAddress | cut -d '"' -f 4
|
1
2
|
# 192.168.0.103 is host ip
docker run -t -d --add-host=docker:192.168.0.103 centos:6.8
|
1
|
docker ps -aq -f status=exited
|
1
|
docker ps -aq --no-trunc | xargs docker rm
|
1
|
docker images -q --filter dangling=true | xargs docker rmi
|
DocStation provides GUI for monitoring, configuring and managing services and containers.